Arrays and Lists are allowed to be used in JSON. If there is no item in the list, an exception will be thrown. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. The below example combines two lists into a single list. How to Install Python Pandas on Windows and Linux? How I handled Editing, Deleting and Adding Quiz Questions in a JSON file for my Simple Quiz written in Python. In this example, we will take a Python list with some numbers in it and convert it to JSON string. In this tutorial, we learned list creation methods. The length of the list increases by one. decimal.Decimal). For example I can have 'xyz', '4444' and 'test2' appear as JSON entries multiple times. English Grammar; School Programming; Mathematics. Familiarity with installing Python 3. Get started, freeCodeCamp is a donor-supported tax-exempt 501(c)(3) charity organization (United States Federal Tax Identification Number: 82-0779546). You should use Insert() when insertion is required at a specific index or range of indexes. Add two numbers. This work is licensed under a Creative Commons Attribution-NonCommercial- ShareAlike 4.0 International License. Python | Pandas Dataframe/Series.head() method, Python | Pandas Dataframe.describe() method, Dealing with Rows and Columns in Pandas DataFrame, Python | Pandas Extracting rows using .loc[], Python | Extracting rows using Pandas .iloc[], Python | Pandas Merging, Joining, and Concatenating, Python | Working with date and time using Pandas, Python | Read csv using pandas.read_csv(), Python | Working with Pandas and XlsxWriter | Set 1. To learn more, see our tips on writing great answers. Styling contours by colour and by line thickness in QGIS. If you have a Python object, you can convert it into a JSON string by So I'm struggling on adding an element to a json list that's saved on my machine. In the image below, "P" is at index "0" whereas "H" is at index "3". You may read about it here. Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff. of indents: You can also define the separators, default value is (", ", ": "), which There are currently 5 . The first index starts at. But we are going to do it anyway because eventually, we are adding something to a list. As you can see above, I had added an if statement and a try-except statement. Check prime number. In this case, the problem of axes of space. Check leap year. Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2, JSON datetime between Python and JavaScript. However, if we are given a sorted list and we are asked to maintain the order of the elements while inserting a new element, it might become a tedious task. Does Counterspell prevent from any further spells being cast on a given turn? GEEK = {'g', . Here is an example of extending a list: As written, the code would append to the JSON_FILE. How I handled Editing, Deleting and Adding Quiz Questions in a JSON file for my Simple Quiz written in Python. Then, I created a simple for loop script just to check if the contents are right in the file I made. Here list is the list you are adding elements to and sequence is any valid sequence (list, tuple, string, range, etc.). Selenium tries to click on the exact center of the element. Where does this (supposedly) Gibson quote come from? Solution 1. JSON (JavaScript Object Notation) is a file that is mainly used to store and transfer data mostly between a server and a web application. I had created this function because I realize for my edit and delete function I needed to select a question. Update Dictionary. Read: Python convert dictionary to an array Python convert Dataframe to list of dictionaries. Get certifiedby completinga course today! Instead of repeating the code inside the edit and delete function, I could just made one function to be reused by the two functions. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. In the upcoming code, we will keep adding elements to the array. I want it so that the new saved json file will be. If you have a Python object, you can convert it into a JSON string by using the json.dumps() method. extend (): extends the list by appending elements from the iterable. Steps for Appending to a JSON File. json_decoded ['students'] is a list of dictionaries that you can simply iterate and update in a loop. to separate keys from values: Use the separators parameter to change the If you have a JSON string, you can parse it by using the Finally, is there a better place to put the JSON piece? Here, I have a Question, A, B, C, and Answer as my keys. I am a DevOps Consultant and writer at FreeCodeCamp. using the json.dumps() method. Lists are one of the most useful and versatile data types available in Python. Simply the extend() method. Not the answer you're looking for? If you read this far, tweet to the author to show them you care. The insert() method inserts an item at the specified index: Note: As a result of the examples above, the lists will now contain 4 items. If we wanted to access the last item from the list above, we could also use index -1: We can easily find the length of a list using the len() method. We can make the above code efficient by completely skipping the for loop - append() combination and using list comprehension instead. Syntax: json.dumps (object) Parameter: It takes Python Object as the parameter. It is one of many methods to add an element to the list. I am thinking of another method to use that will be better but for now this is what we have that works. One cell in Colab to handle reading and writing codes for the JSON file. In this example, we will take a Python List with Dictionaries as elements and convert it to JSON string. A relative import is resolved relative to the importing file and cannot resolve to an ambient module declaration. Example 2: Updating a JSON file. We can modify our previous example to achieve list comprehension. Methods to Add Items to a List. import json with open ('file.json', 'a') as outfile: outfile.write (json.dumps (data)) outfile.write (",") outfile.close () View another examples Add Own solution. Python supports JSON through a built-in package called JSON. Syntax: dict.update([other])Parameters: Takes another dictionary or an iterable key/value pair.Return type: Returns None. The append() is a list method in python that adds an element to the end of the list. Unlike the append() method which adds elements only at the end of the list, the insert() method adds an element at a specific index of the list. To use this feature, we import the JSON package in Python script. Extending other sequences like strings, range, etc. It is also known as a sequence or array in other programming languages. Is it correct to use "the" before "materials used in making buildings are"? "Parse JSON" action transforms the Json structure into an array, and "Filter Array" selects elements of the array that match the given criteria (where myDay is equal to "day" in your case). The main points worth mentioning: Thanks for contributing an answer to Stack Overflow! Linear regulator thermal information missing in datasheet. Number System; Algebra; . The text in JSON is done through quoted-string which contains the value in key-value mapping within { }. We also looked at some examples along with a practical implementation of stacks to see how it all works. Why do small African island nations perform better than African continental nations, considering democracy and human development? The index is the position of the element you want to add to the list. By using our site, you However I'm getting the error of "JSONDecodeError: Expecting value: line 1 column 1 (char 0)". list.append () - always adds items (strings, numbers, lists) at the end of the list. List comprehension makes the code simple and readable by combining the for loop and append() into a single line. Note that dump () takes two positional arguments: (1) the data object to be serialized, and (2) the file-like object to which the bytes will be written. The push() method appends an item to the list. Method 1: Appending a dictionary to a list with the same key and different values. Check if string contains substring in Python, Python replace character in string by index, Python list comprehension multiple conditions, Paraphrasing Tools Use NLP Libraries in Python. The update () method will update the dictionary with the items from a given argument. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. I had learned alot from this quiz exercise and actually discovered how useful creating functions are in a project since you can reuse them again and again. I will leave the explanation of JSON file to the experts. Updated on September 20, 2022, Simple and reliable cloud website hosting, "Please enter a number to add to list:\n", New! update (): This method updates the dictionary with elements from another dictionary object or from an iterable key/value pair. How do I make a flat list out of a list of lists? An element can be a number, string, list, dictionary, tuple, or even another list. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. How to append a new row to an existing csv file? In practice, you can use .append() to add any kind of object to a given list: Is there a way to correct this? Example below shows converting JSON string to a dictionary which is done by: Arrays and Lists are allowed to be used in JSON. We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. What I'm trying to is the json will update with the user's typed message. To add an item to the end of the list, use the append() See details of the pop() method here. lists, you can add any iterable object (tuples, sets, dictionaries W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. I can access each of the elements, but I do not know how to loop over them to add them into a dataframe or another appropriate format maybe like dict. You can do the following in order to manipulate the dict the way you want: for s in json_decoded [ 'students' ]: s [ 'country'] = 'UK'. Example. So you shouldn't need to grab the element at index "0" if there is no index 0. The __len__ method determines the length of the stack. Example below show simple converting of JSON file to dictionary using method from module JSON: Sometimes the JSON is received in string format. If it is not in the list, then add it to the list. If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: W3Schools is optimized for learning and training. What sort of strategies would a medieval military use against a fantasy giant? Python Program. This function made me fall in love with the great use of creating functions for things you may use repeatedly. default separator: The json.dumps() method has parameters to This function takes a list as an argument and returns a JSON string. Let's call the class functions and see the output in action. You can make a tax-deductible donation here. For this article though, I will be mostly discussing about how I actually handles the Editing, Deleting and Adding of questions in my program. Adds its argument as a single element to the end of a list. It means that a script (executable) file which is made of text in a programming language, is used to store and transfer the data. Replacing broken pins/legs on a DIP IC package. Python JSON to list. I can't figure out what I'm doing wrong, any help would be appreciated. Is there a single-word adjective for "having exceptionally strong moral principles"? Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2. Preview style <!-- Changes that affect Black's preview style --> - Enforce empty lines before classes and functions w. Why does Mister Mxyzptlk need to have a weakness in the comics? @JD2775, Yep, you should be careful you restart your kernel (if running in Jupyter notebook) to make sure your variables aren't stale; or make sure you reset your results list via, Best way to add dictionary entry and append to JSON file in Python, How Intuit democratizes AI development across teams through reusability. Python Script Examples Resources Event Scheduler Summary Scripting is a very smart and useful programming discipline but its terms can also be confusing and mystifying. Asking for help, clarification, or responding to other answers. As you remember in my previous post, I posted a quiz testing my friends and our friendship using the program I made in python. Notice the commented out lines here: Both of the methods for filling an empty list are valid and suitable in different scenarios. We can leave them empty and supply values later in the program: We can also provide values while creating a list: This would create a list as shown in the image below: As list items are ordered, you can access them using their index. It is a collection of items, which are separated by commas. Learn more. We also have thousands of freeCodeCamp study groups around the world. Does a summoned creature play immediately after being summoned by a ready action? Must be able to create, read and write on a JSON file. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. Maybe adjust some codes to make it more efficient. Let's create a stack class where we first declare an empty list in the init method. We want to add another JSON data after emp_details. Here we are going to append a dictionary of integer type to an empty list using for loop with same key but different values. But if you need to make multiple additions, extend() is a better option as it adds iterable items in one batch. Creating a JSON response using Django and Python, Posting a File and Associated Data to a RESTful WebService preferably as JSON, How to create dictionary and add key value pairs dynamically in Javascript. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, How was the file saved? parse_int, if specified, will be called with the string of every JSON int to be decoded.By default, this is equivalent to int(num_str). Syntax: json.loads(json_string)Parameter: It takes JSON string as the parameter.Return type: It returns the python dictionary object. Convert from Python to JSON. Find centralized, trusted content and collaborate around the technologies you use most. Append() always adds a single item at the end of a list. I tried something like results = [ {"name": i, "children": j} for i,j in results.items ()]. List Concatenation: We can use the + operator to concatenate multiple lists and create a new list. The same can also be achieved by using the + operator. Let's discuss certain way in which this problem can be solved. Find the factorial of a number. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. These JSON files are the SSOT of everything there is to know about Redis commands, and it is reflected fully in COMMAND INFO. The main points worth mentioning: Use can use a list to hold your arguments and use *args to unpack when you supply them to add_entry. I simply wanna challenge myself into using a format that I had never used before and gain some new experience. 2) To overwrite the output file, you need to close the file first and reopen it. Delete the hardcoded command table and replace it with an auto-generated table, based on a JSON file that describes the commands (each command must have a JSON file). Changelog 22.12. C++ Programming - Beginner to Advanced; Java Programming - Beginner to Advanced; C Programming - Beginner to Advanced; Android App Development with Kotlin(Live) Web Development. This time, I needed to revise the entire quiz to fit the requirements as given by our instructor. Honestly, I think this one needs to be improved on how I convert my user input into a dictionary that I can then append to my JSON string. Python add element to json object code example. To convert a Python List to JSON, use json.dumps() function. List. The colorMode()function is used to change the numerical range used for specifying colors and to switch color systems. For writing to JSON, now you have a list, you can simply iterate your list and write in one function at the end. We know how to create the list and update the list by various built-in methods of the list. Because it extends the list by adding each element of the list to another list as its own element. insert (): inserts the element before the given index. I still need to work on some adjustments for this project. . An overview of lists and how they are defined. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, this is very helpful, thank you for taking the time to explain it and code a solution for me. ; To check / avoid duplicates, you can use set to track items already added. Return type: It returns the JSON string. In the Python Programming Language, a list can be accessed either by index or slice operator. Python Programming Foundation -Self Paced Course, Python - Difference between json.dump() and json.dumps(), Python - Difference Between json.load() and json.loads(), Python - Append content of one text file to another. Free Python Complete Course for Beginners in Urdu Go to course home . Explore Python Examples Reference Materials. Is there a single-word adjective for "having exceptionally strong moral principles"? 0 . Our mission: to help people learn to code for free. As written, the code would append to the JSON_FILE.
python json add element to list
- Post author:
- Post published:March 17, 2023
- Post category:new orleans burlesque show 2021
python json add element to listYou Might Also Like
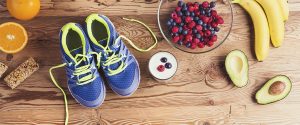
python json add element to listoxford ring road map
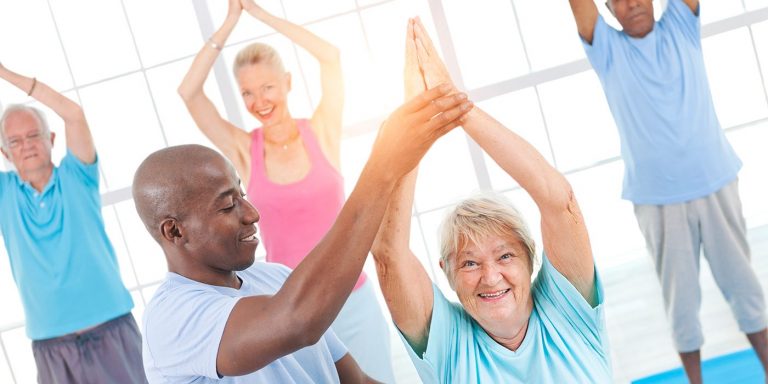
python json add element to listbranford house winter wedding
