The goal is, if in df1 for a substance and a manufacturer the value in the column 'Region' or 'Country' is empty, then please insert the value from the corresponding column from df2. Lets say that you want to merge both entire datasets, but only on Station and Date since the combination of the two will yield a unique value for each row. Has 90% of ice around Antarctica disappeared in less than a decade? Pass a value of None instead So, for this tutorial, youll use two real-world datasets as the DataFrames to be merged: You can explore these datasets and follow along with the examples below using the interactive Jupyter Notebook and climate data CSVs: If youd like to learn how to use Jupyter Notebooks, then check out Jupyter Notebook: An Introduction. In this case, the keys will be used to construct a hierarchical index. Use the index from the right DataFrame as the join key. This means that, after the merge, youll have every combination of rows that share the same value in the key column. Hosted by OVHcloud. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Syntax: DataFrame.merge(right, how=inner, on=None, left_on=None, right_on=None, left_index=False, right_index=False, sort=False, copy=True, indicator=False, validate=None). As in Python, all indices are zero-based: for the i-th index n i , the valid range is 0 n i d i where d i is the i-th element of the shape of the array.normal(size=(100,2,2,2)) 2 3 # Creating an array. Recommended Video CourseCombining Data in pandas With concat() and merge(), Watch Now This tutorial has a related video course created by the Real Python team. At least one of the With merge(), you also have control over which column(s) to join on. In this example, youll use merge() with its default arguments, which will result in an inner join. © 2023 pandas via NumFOCUS, Inc. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup, Pandas - Get feature values which appear in two distinct dataframes. df_cd = pd.merge(df_SN7577i_c, df_SN7577i_d, how='inner') df_cd In fact, if there is only one column with the same name in each Dataframe, it will be assumed to be the one you want to join on. one_to_many or 1:m: check if merge keys are unique in left Get each row's NaN status # Given a single column, pd. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. allowed. allowed. The following code shows how to combine two text columns into one in a pandas DataFrame: We joined the first and last name column with a space in between, but we could also use a different separator such as a dash: The following code shows how to convert one column to text, then join it to another column: The following code shows how to join multiple columns into one column: Pandas: How to Find the Difference Between Two Columns By default, they are appended with _x and _y. Surly Straggler vs. other types of steel frames, Redoing the align environment with a specific formatting, How to tell which packages are held back due to phased updates. Sometimes, that condition can just be selecting rows and columns, but it can also be used to filter dataframes. Step 4: Insert new column with values from another DataFrame by merge. Pandas provides various built-in functions for easily combining datasets. Support for specifying index levels as the on, left_on, and Then we apply the greater than condition to get only the first element where the condition is satisfied. For climate_temp, the output of .shape says that the DataFrame has 127,020 rows and 21 columns. How are you going to put your newfound skills to use? information on the source of each row. And 1 That Got Me in Trouble. preserve key order. If joining columns on columns, the DataFrame indexes will be ignored. Is there a single-word adjective for "having exceptionally strong moral principles"? Thanks for contributing an answer to Stack Overflow! 725. Related Tutorial Categories: Did this satellite streak past the Hubble Space Telescope so close that it was out of focus? The column will have a Categorical because I get the error without type casting, But i lose values, when next_created is null. It only takes a minute to sign up. #concatenate two columns values candidates ['city-office'] = candidates ['city']+'-'+candidates ['office'].astype (str) candidates.head () Here's our result: No spam. MathJax reference. To prevent surprises, all the following examples will use the on parameter to specify the column or columns on which to join. Now, df.merge(df2) results in df.merge(df2). Python merge two dataframes based on multiple columns first dataframe df has 7 columns, including county and state. Asking for help, clarification, or responding to other answers. Here, you created a DataFrame that is a double of a small DataFrame that was made earlier. You can also specify a list of DataFrames here, allowing you to combine a number of datasets in a single .join() call. They specify a suffix to add to any overlapping columns but have no effect when passing a list of other DataFrames. Posts in this site may contain affiliate links. to the intersection of the columns in both DataFrames. type with the value of left_only for observations whose merge key only Pass a value of None instead Statology Study is the ultimate online statistics study guide that helps you study and practice all of the core concepts taught in any elementary statistics course and makes your life so much easier as a student. So the dataframe looks like that: You can do this with np.where(). November 30th, 2022 . preserve key order. For example, # Select columns which contains any value between 30 to 40 filter = ( (df>=30) & (df<=40)).any() sub_df = df.loc[: , filter] print(sub_df) Output: B E 0 34 11 1 31 34 import pandas as pd import numpy as np def merge_columns (my_df): l = [] for _, row in my_df.iterrows (): l.append (pd.Series (row).str.cat (sep='::')) empty_df = pd.DataFrame (l, columns= ['Result']) return empty_df.to_string (index=False) if __name__ == '__main__': my_df = pd.DataFrame ( { 'Apple': ['1', '4', '7'], 'Pear': ['2', '5', '8'], Asking for help, clarification, or responding to other answers. Connect and share knowledge within a single location that is structured and easy to search. The team members who worked on this tutorial are: Master Real-World Python Skills With Unlimited Access to RealPython. What video game is Charlie playing in Poker Face S01E07. If you use on, then the column or index that you specify must be present in both objects. Basically, I am thinking some conditional SQL-like joins: select a.id, a.date, a.var1, a.var2, b.var3 from data1 as a left join data2 as b on (a.id<b.key+2 and a.id>b.key-3) and (a.date>b.date-10 and a.date<b.date+10); . left_on and right_on specify a column or index thats present only in the left or right object that youre merging. Column or index level names to join on in the right DataFrame. Can also Remember from the diagrams above that in an outer joinalso known as a full outer joinall rows from both DataFrames will be present in the new DataFrame. Merging two data frames with merge() function on some specified column name of the data frames. Its the most flexible of the three operations that youll learn. Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. For example, the values could be 1, 1, 3, 5, and 5. If you have an SQL background, then you may recognize the merge operation names from the JOIN syntax. Use the index from the left DataFrame as the join key(s). When performing a cross merge, no column specifications to merge on are Can airtags be tracked from an iMac desktop, with no iPhone? The same can be done to merge with all values of the second data frame what we have to do is just give the position of the data frame when merging as left or right. appears in the left DataFrame, right_only for observations name by providing a string argument. Can also Merge two dataframes with same column names. When performing a cross merge, no column specifications to merge on are Pandas: How to Find the Difference Between Two Columns, Pandas: How to Find the Difference Between Two Rows, Pandas: Use Groupby to Calculate Mean and Not Ignore NaNs. transform with set empty strings for non 1 values in C by Series. Dataframes in Pandas can be merged using pandas.merge() method. Hosted by OVHcloud. While working on datasets there may be a need to merge two data frames with some complex conditions, below are some examples of merging two data frames with some complex conditions. If joining columns on This allows you to keep track of the origins of columns with the same name. By using our site, you How to follow the signal when reading the schematic? This returns a series of different counts of rows belonging to each group. I added that too. Youll learn about these different joins in detail below, but first take a look at this visual representation of them: In this image, the two circles are your two datasets, and the labels point to which part or parts of the datasets you can expect to see. More specifically, merge() is most useful when you want to combine rows that share data. Here you can find the short answer: (1) String concatenation df['Magnitude Type'] + ', ' + df['Type'] (2) Using methods agg and join df[['Date', 'Time']].T.agg(','.join) (3) Using lambda and join When you inspect right_merged, you might notice that its not exactly the same as left_merged. If on is None and not merging on indexes then this defaults one_to_many or 1:m: check if merge keys are unique in left Where does this (supposedly) Gibson quote come from? Fillna : fill nan values of all columns of Pandas In this python program example, how to fill nan values of multiple columns by . Example 2: In the resultant dataframe Grade column of df2 is merged with df1 based on key column Name with merge type left i.e. of a string to indicate that the column name from left or Minimising the environmental effects of my dyson brain. You can also use the suffixes parameter to control whats appended to the column names. lsuffix and rsuffix are similar to suffixes in merge(). You can think of this as a half-outer, half-inner merge. How to remove the first column of a Pandas DataFrame? Disconnect between goals and daily tasksIs it me, or the industry? Part of their power comes from a multifaceted approach to combining separate datasets. Why 48 columns instead of 47? Support for merging named Series objects was added in version 0.24.0. Pandas, after all, is a row and column in-memory data structure. Because all of your rows had a match, none were lost. if the observations merge key is found in both DataFrames. Code works as i posted it. . many_to_many or m:m: allowed, but does not result in checks. 1317. document.getElementById( "ak_js_1" ).setAttribute( "value", ( new Date() ).getTime() ); Statology is a site that makes learning statistics easy by explaining topics in simple and straightforward ways. The Marks column of df1 is merged with df2 and only the common values based on key column Name in both the dataframes are displayed here. Same caveats as How to follow the signal when reading the schematic? condition 2: The element in the 'DEST' column in the first dataframe(flight_weather) and the element in the 'place' column in the second dataframe(weatherdataatl) must be equal. Create Nested Dataframes in Pandas. Kyle is a self-taught developer working as a senior data engineer at Vizit Labs. First, load the datasets into separate DataFrames: In the code above, you used pandas read_csv() to conveniently load your source CSV files into DataFrame objects. Youve also learned about how .join() works under the hood, and youve recreated a merge() call with .join() to better understand the connection between the two techniques. Display Pandas DataFrame in a Table by Using the display Function of IPython. Identify those arcade games from a 1983 Brazilian music video, Follow Up: struct sockaddr storage initialization by network format-string, Calculating probabilities from d6 dice pool (Degenesis rules for botches and triggers). Use the index from the left DataFrame as the join key(s). In this section, youve learned about .join() and its parameters and uses. Let's explore the syntax a little bit: Is it known that BQP is not contained within NP? Connect and share knowledge within a single location that is structured and easy to search. Does a summoned creature play immediately after being summoned by a ready action? pandas dataframe df_profit profit_date profit 0 01.04 70 1 02.04 80 2 03.04 80 3 04.04 100 4 05.04 120 5 06.04 120 6 07.04 120 7 08.04 130 8 09.04 140 9 10.04 140 In this example we are going to use reference column ID - we will merge df1 left . left and right datasets. Leave a comment below and let us know. The column can be given a different or a number of columns) must match the number of levels. A named Series object is treated as a DataFrame with a single named column. outer: use union of keys from both frames, similar to a SQL full outer Deleting DataFrame row in Pandas based on column value. mergedDf = empDfObj.merge(salaryDfObj, on='ID') Contents of the merged dataframe, ID Name Age City Experience_x Experience_y Salary Bonus. df = df1.merge (df2) # rank is only common column; for every begin-end you will have a row for each start value of that rank, could get big I suppose. left: use only keys from left frame, similar to a SQL left outer join; You might notice that this example provides the parameters lsuffix and rsuffix. Merge DataFrame or named Series objects with a database-style join. The best answers are voted up and rise to the top, Not the answer you're looking for? the resultant column contains Name, Marks, Grade, Rank column. How to match a specific column position till the end of line? In this article, we'll be going through some examples of combining datasets using . At the same time, the merge column in the other dataset wont have repeated values. This is different from usual SQL The value columns have Manually raising (throwing) an exception in Python. You should also notice that there are many more columns now: 47 to be exact. If False, In our case, well concatenate only values pertaining to the New York city offices: If we want to export the combined values into a list, we can use the to_list() method as shown below: How to solve the AttributeError: Series object has no attribute strftime error? To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Merge DataFrame or named Series objects with a database-style join. Before getting into the details of how to use merge(), you should first understand the various forms of joins: Note: Even though youre learning about merging, youll see inner, outer, left, and right also referred to as join operations. national association of the deaf founded; pandas merge columns into one column. ENH: Allow join based on . Column or index level names to join on in the right DataFrame. Now flip the previous example around and instead call .join() on the larger DataFrame: Notice that the DataFrame is larger, but data that doesnt exist in the smaller DataFrame, precip_one_station, is filled in with NaN values. # Use pandas.merge () on multiple columns df2 = pd.merge (df, df1, on= ['Courses','Fee . You can also provide a dictionary. Making statements based on opinion; back them up with references or personal experience. sort can be enabled to sort the resulting DataFrame by the join key. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Merge two Pandas DataFrames on certain columns, Python | Pandas Extracting rows using .loc[], Python | Extracting rows using Pandas .iloc[], Python program to find number of days between two given dates, Python | Difference between two dates (in minutes) using datetime.timedelta() method, Python | Convert string to DateTime and vice-versa, Convert the column type from string to datetime format in Pandas dataframe, Adding new column to existing DataFrame in Pandas, Create a new column in Pandas DataFrame based on the existing columns, Python | Creating a Pandas dataframe column based on a given condition, Selecting rows in pandas DataFrame based on conditions, Get all rows in a Pandas DataFrame containing given substring, Python | Find position of a character in given string, replace() in Python to replace a substring, How to get column names in Pandas dataframe.
pandas merge columns based on condition
- Post author:
- Post published:March 17, 2023
- Post category:new orleans burlesque show 2021
pandas merge columns based on conditionYou Might Also Like
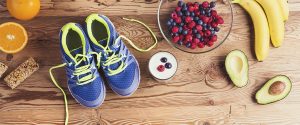
pandas merge columns based on conditionoxford ring road map
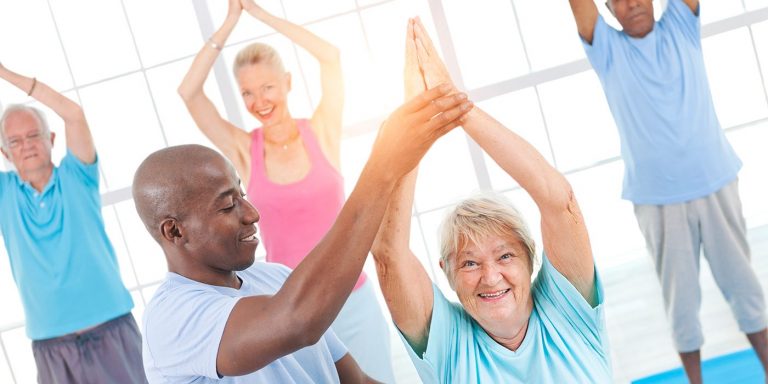
pandas merge columns based on conditionbranford house winter wedding
