Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2. This method adds a counter to an iterable and returns them together as an enumerated object. (Uglier but works for what you're trying to do. FOR Loops are one of them, and theyre used for sequential traversal. Changelog 2.3.0 What's Changed * Fix missing URL import for the Stream class example in README by hiohiohio in https . "readability counts" The speed difference in the small <1000 range is insignificant. While iterating over a sequence you can also use the index of elements in the sequence to iterate, but the key is first to calculate the length of the list and then iterate over the series within the range of this length. How can I access environment variables in Python? Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. @Georgy makes sense, on python 3.7 enumerate is total winner :). It used a generator function which allows the last value of the index variable to be repeated. Get tutorials, guides, and dev jobs in your inbox. from last row to row at 0th index. Using Kolmogorov complexity to measure difficulty of problems? They execute depending on the conditions of the current cycle. Syntax DataFrameName.set_index ("column_name_to_setas_Index",inplace=True/False) where, inplace parameter accepts True or False, which specifies that change in index is permanent or temporary. vegan) just to try it, does this inconvenience the caterers and staff? 'fee_pct': 0.50, 'platform': 'mobile' } Method 1: Iteration Using For Loop + Indexing The easiest way to iterate through a dictionary in Python, is to put it directly in a for loop. This enumerate object can be easily converted to a list using a list() constructor. A for loop most commonly used loop in Python. timeit ( for_loop) 267.0804728891719. There are ways, but they'd be tricky to say the least. A for loop is faster than a while loop. Your email address will not be published. The same loop is written as a list comprehension looks like: Change value of the currently iterated element in the list example. In Python, the for loop is used to run a block of code for a certain number of times. It returns a zip object - an iterator of tuples in which the first item in each passed iterator is paired together, the second item in each passed iterator is paired together, and analogously for the rest of them: The length of the iterator that this function returns is equal to the length of the smallest of its parameters. Here we are accessing the index through the list of elements. You can use continuekeyword to make the thing same: @Someone \i is the height of the horizontal sections in the boxing bag and \kare the angles of the radius (the three dashed lines). If I were to iterate nums = [1, 2, 3, 4, 5] I would do. This means that no matter what you do inside the loop, i will become the next element. Why are Suriname, Belize, and Guinea-Bissau classified as "Small Island Developing States"? Making statements based on opinion; back them up with references or personal experience. List comprehension will make a list of the index and then gives the index and index values. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. It is the counter from which indexing will start. In this article, we will discuss how to access index in python for loop in Python. These two-element lists were constructed by passing pairs to the list() constructor, which then spat an equivalent list. This allows you to reference the current index using the loop variable. The standard way of dealing with this is to completely exhaust the divisions by i in the body of the for loop itself: It's slightly more efficient to do the division and remainder in one step: The only way to change the next value yielded is to somehow tell the iterable what the next value to yield should be. Find centralized, trusted content and collaborate around the technologies you use most. What we did in this example was enumerate every value in a list with its corresponding index, creating an enumerate object. Python | Change column names and row indexes in Pandas DataFrame, Change Data Type for one or more columns in Pandas Dataframe. What does the ** operator mean in a function call? Linear Algebra - Linear transformation question, The difference between the phonemes /p/ and /b/ in Japanese. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. In the loop, you set value equal to the item in values at the current value of index. This method adds a counter to an iterable and returns them together as an enumerated object. Often when you're trying to loop with indexes in Python, you'll find that you actually care about counting upward as you're looping, not actual indexes. We can access an item of a tuple by using its index number inside the index operator [] and this process is called "Indexing". Because of this, we usually don't really need indices of a list to access its elements, however, sometimes we desperately need them. This includes any object that could be a sequence (string, tuples) or a collection (set, dictionary). You can give any name to these variables. Print the required variables inside the for loop block. iDiTect All rights reserved. We can access the index in Python by using: Using index element Using enumerate () Using List Comprehensions Using zip () Using the index elements to access their values The index element is used to represent the location of an element in a list. Your email address will not be published. They are available in Python by importing the array module. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. The easiest way to fix your code is to iterate over the indexes: Thanks for contributing an answer to Stack Overflow! Mutually exclusive execution using std::atomic? Where was Data Visualization in Python with Matplotlib and Pandas is a course designed to take absolute beginners to Pandas and Matplotlib, with basic Python knowledge, and 2013-2023 Stack Abuse. Share Follow answered Feb 9, 2013 at 6:12 Volatility 30.6k 10 80 88 4 Is this the only way? To change the index values we need to use the set_index method which is available in pandas allows specifying the indexes. To get these indexes from an iterable as you iterate over it, use the enumerate function. How to change index of a for loop Suppose you have a for loop: for i in range ( 1, 5 ): if i is 2 : i = 3 The above codes don't work, index i can't be manually changed. Why was a class predicted? As we access the list by "i", "i" is formatted as the item price (or whatever it is). Here we will also cover the below examples: A for loop in Python is used to iterate over a sequence (such as a list, tuple, or string) and execute a block of code for each item in the sequence. In this article, we will discuss how to access index in python for loop in Python. Let's create a series: Python3 Why is there a voltage on my HDMI and coaxial cables? Catch multiple exceptions in one line (except block). Therefore, whatever changes you make to the for loop variable get effectively destroyed at the beginning of each iteration. Even if you changed the value, that would not change what was the next element in that list. What is the point of Thrower's Bandolier? acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe, Python program to convert a list to string, Reading and Writing to text files in Python, Different ways to create Pandas Dataframe, isupper(), islower(), lower(), upper() in Python and their applications, Python | Program to convert String to a List, Check if element exists in list in Python, How to drop one or multiple columns in Pandas Dataframe, Draw Black Spiral Pattern Using Turtle in Python, Python Flags to Tune the Behavior of Regular Expressions. Required fields are marked *. How to get the index of the current iterator item in a loop? Note that zip with different size lists will stop after the shortest list runs out of items. @BrenBarn some times messy is the only way, @BrenBarn, it is very common in other languages; but, yes, I've had numerous bugs because of it, Great details. In this tutorial, you will learn how to use Python for loop with index. So, in this section, we understood how to use the map() for accessing the Python For Loop Index. Changelog 22.12. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Connect and share knowledge within a single location that is structured and easy to search. With a lot of standard iterables, this isn't possible. You can access the index even without using enumerate (). Python program to Increment Numeric Strings by K, Ways to increment Iterator from inside the For loop in Python, Python program to Increment Suffix Number in String. In the above example, the code creates a list named new_str2 with the values [Germany, England, France]. Check out my profile. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe, Python program to convert a list to string, Reading and Writing to text files in Python, Different ways to create Pandas Dataframe, isupper(), islower(), lower(), upper() in Python and their applications, Python | Program to convert String to a List, Check if element exists in list in Python, How to drop one or multiple columns in Pandas Dataframe, How to add time onto a DateTime object in Python, Predicting Stock Price Direction using Support Vector Machines. import timeit # A for loop example def for_loop(): for number in range(10000) : # Execute the below code 10000 times sum = 3+4 #print (sum) timeit. You can also access items from their negative index. Changelog 3.28.0 -------------------- Features ^^^^^^^^ - Support provision of tox 4 with the ``min_version`` option - by . Basic Syntax of a For Loop in Python. The zip function can be used to iterate over multiple sequences in parallel, allowing you to reference the corresponding items at each index. Does Counterspell prevent from any further spells being cast on a given turn? Now that we've explained how this function works, let's use it to solve our task: In this example, we passed a sequence of numbers in the range from 0 to len(my_list) as the first parameter of the zip() function, and my_list as its second parameter. enumerate () method is the most efficient method for accessing the index in a for loop. This method combines indices to iterable objects and returns them as an enumerated object. The while loop has no such restriction. Example 1: Incrementing the iterator by 1. Connect and share knowledge within a single location that is structured and easy to search. Let's change it to start at 1 instead: A list comprehension is a way to define and create lists based on already existing lists. I have been working with Python for a long time and I have expertise in working with various libraries on Tkinter, Pandas, NumPy, Turtle, Django, Matplotlib, Tensorflow, Scipy, Scikit-Learn, etc I have experience in working with various clients in countries like United States, Canada, United Kingdom, Australia, New Zealand, etc. What video game is Charlie playing in Poker Face S01E07? Using While loop: We can't directly increase/decrease the iteration value inside the body of the for loop, we can use while loop for this purpose. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Fruit at 3rd index is : grapes. Loop variable index starts from 0 in this case. Python For loop is used for sequential traversal i.e. Hi. The for loops in Python are zero-indexed. It handles nested loops better than the other examples. The for loop accesses the "listos" variable which is the list. When you use a var in a for loop like this, you can a read-write copy of the number value but it's not bound to the original numbers array. Python3 for i in range(5): print(i) Output: 0 1 2 3 4 Example 2: Incrementing the iterator by an integer value n. Python3 n = 3 for i in range(0, 10, n): print(i) Output: 0 3 6 9 # i.e. The for loop variable can be changed inside each loop iteration, like this: It does get modified inside each for loop iteration. The count seems to be more what you intend to ask for (as opposed to index) when you said you wanted from 1 to 5. the initialiser "counter" is used for item number. also, if you are modifying elements in a list in the for loop, you might also need to update the range to range(len(list)) at the end of each loop if you added or removed elements inside it. I'm writing something like an assembly code interpreter. Is this the only way? Is it correct to use "the" before "materials used in making buildings are"? var d = new Date() The zip method in Python is used to zip the index and values at a time, we have to pass two lists one list is of index elements and another list is of elements. so after you do your special attribute{copy paste} you can still edit the indentation. Asking for help, clarification, or responding to other answers. They differ in when and why they execute. Then range () creates an iterator running from the default starting value of 0 until it reaches len (values) minus one. The easiest, and most popular method to access the index of elements in a for loop is to go through the list's length, increasing the index. Although I started out using enumerate, I switched to this approach to avoid having to write logic to select which object to enumerate. Nonetheless, this is how I implemented it, in a way that I felt was clear what was happening. If you want to properly keep track of the "index value" in a Python for loop, the answer is to make use of the enumerate() function, which will "count over" an iterableyes, you can use it for other data types like strings, tuples, and dictionaries.. We can see below that enumerate() doesn't give us the desired result: We can access the indices of a pandas Series in a for loop using .items(): You can use range(len(some_list)) and then lookup the index like this, Or use the Pythons built-in enumerate function which allows you to loop over a list and retrieve the index and the value of each item in the list. enumerate() is a built-in Python function which is very useful when we want to access both the values and the indices of a list. This PR updates black from 19.10b0 to 23.1a1. We can achieve the same in Python with the following . It adds a new column index_column with index values to DataFrame.. You'd probably wanna assign i to another variable and alter it. And when building new apps we will need to choose a backend to go with Angular. It can be achieved with the following code: Here, range(1, len(xs)+1); If you expect the output to start from 1 instead of 0, you need to start the range from 1 and add 1 to the total length estimated since python starts indexing the number from 0 by default. By using our site, you This will break down if there are repeated elements in the list as. When we want to retrieve only particular columns instead of all columns follow the below code, Python Programming Foundation -Self Paced Course, Change the order of index of a series in Pandas, Python | Pandas Series.nonzero() to get Index of all non zero values in a series, Get minimum values in rows or columns with their index position in Pandas-Dataframe, Mapping external values to dataframe values in Pandas, Highlight the negative values red and positive values black in Pandas Dataframe, PyQt5 - Change the item at specific index in ComboBox. When the values in the array for our for loop are sequential, we can use Python's range () function instead of writing out the contents of our array. Is it correct to use "the" before "materials used in making buildings are"? How to get the Iteration index in for loop in Python. It's worth noting that this is the fastest and most efficient method for acquiring the index in a for loop. How Intuit democratizes AI development across teams through reusability. The index () method is almost the same as the find () method, the only difference is that the find () method returns -1 if the value is not found. Using a for loop, iterate through the length of my_list. Is "pass" same as "return None" in Python? The basic syntax or the formula of for loops in Python looks like this: for i in data: do something i stands for the iterator. How Intuit democratizes AI development across teams through reusability. You can get the values of that column in order by specifying a column of pandas.DataFrame and applying it to a for loop. Python arrays are homogenous data structure. If you preorder a special airline meal (e.g. To break these examples down, say we have a list of items that we want to iterate over with an index: Now we pass this iterable to enumerate, creating an enumerate object: We can pull the first item out of this iterable that we would get in a loop with the next function: And we see we get a tuple of 0, the first index, and 'a', the first item: we can use what is referred to as "sequence unpacking" to extract the elements from this two-tuple: and when we inspect index, we find it refers to the first index, 0, and item refers to the first item, 'a'. Changing the index permanently by specifying inplace=True in set_index method. Loop variable index starts from 0 in this case. Full Stack Development with React & Node JS(Live) Java Backend . It is used to iterate over a sequence (list, tuple, string, etc.) It executes everything in the code block. Programming languages start counting from 0; don't forget that or you will come across an index-out-of-bounds exception. The function paired up each index with its corresponding value, and we printed them as tuples using a for loop. It uses the method of enumerate in the selected answer to this question, but with list comprehension, making it faster with less code. You can use continue keyword to make the thing same: for i in range ( 1, 5 ): if i == 2 : continue A loop with a "counter" variable set as an initialiser that will be a parameter, in formatting the string, as the item number. it is used for iterating over an iterable like String, Tuple, List, Set or Dictionary. TRY IT! Explanation As we didnt specify inplace parameter in set_index method, by default it is taken as false and considered as a temporary operation. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. It's worth noting that this is the fastest and most efficient method for acquiring the index in a for loop. There are 4 ways to check the index in a for loop in Python: The enumerate function is one of the most convenient and readable ways to check the index in for loop when iterating over a sequence in Python. Finally, you print index and value. enumerate() is mostly used in for loops where it is used to get the index along with the corresponding element over the given range. Is it plausible for constructed languages to be used to affect thought and control or mold people towards desired outcomes? What is the purpose of this D-shaped ring at the base of the tongue on my hiking boots? Better is to enclose index inside parenthesis pairs as (index), it will work on both the Python versions 2 and 3. Even though it's a faster way to do it compared to a generic for loop, we should generally avoid using it if the list comprehension itself becomes far too complicated. This kind of indexing is common among modern programming languages including Python and C. If you want your loop to span a part of the list, you can use the standard Python syntax for a part of the list. Using a While Loop. for age in df['age']: print(age) # 24 # 42. source: pandas_for_iteration.py. rev2023.3.3.43278. vegan) just to try it, does this inconvenience the caterers and staff? But they are different from arrays because they are not bound to any specific type. So, in this section, we understood how to use the enumerate() for accessing the Python For Loop Index. Notify me of follow-up comments by email. Not the answer you're looking for? Not the answer you're looking for? Unlike, JavaScript, C, Java, and many other programming languages we don't have traditional C-style for loops. Update flake8 from 3.7.9 to 6.0.0. strftime(): from datetime to readable string, Read specific lines from a file by line number, Split strings into words with multiple delimiters, Conbine items in a list to a single string, Check if multiple strings exist in another string, Check if string exists in a list of strings, Convert string representation of list to a list, Sort list based on values from another list, Sort a list of objects by an attribute of the objects, Get all possible combinations of a list's elements, Get the Cartesian product of a series of lists, Find the cumulative sum of numbers in a list, Extract specific element from each sublist, Convert a String representation of a Dictionary to a dictionary, Create dictionary with dict comprehension and iterables, Filter dictionary to contain specific keys, Python Global Variables and Global Keyword, Create variables dynamically in while loop, Indefinitely Request User Input Until a Valid Response, Python ImportError and ModuleNotFoundError, Calculate Euclidean distance btween two points, Resize an image and keep its aspect ratio, How to indent the contents of a multi-line string in Python, How to Read User Input in Python with the input() function. Got an idea? These for loops are also featured in the C++ . By using our site, you The above codes don't work, index i can't be manually changed. . The tutorial consists of these content blocks: 1) Example Data & Software Libraries 2) Example: Iterate Over Row Index of pandas DataFrame Python will automatically treat transaction_data as a dictionary and allow you to iterate over its keys. Syntax list .index ( elmnt ) Parameter Values More Examples Example What is the position of the value 32: fruits = [4, 55, 64, 32, 16, 32] x = fruits.index (32) Try it Yourself Note: The index () method only returns the first occurrence of the value. The loop variable, also known as the index, is used to reference the current item in the sequence. If we can edit the number by accessing the reference of number variable, then what you asked is possible. How to output an index while iterating over an array in python. 1.1 Syntax of enumerate () rev2023.3.3.43278. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. I would like to change the angle \k of the sections which are plotted with: Pass two loop variables index and val in the for loop. My code is GPL licensed, can I issue a license to have my code be distributed in a specific MIT licensed project? Note: As tuples are ordered sequences of items, the index values start from 0 to the tuple's length. For loop with raw_input, If-statement should increase input by 1, Could not exit a for .. in range.. loop by changing the value of the iterator in python. The zip() function accepts two or more parameters, which all must be iterable. You can simply use a variable such as count to count the number of elements in the list: To print a tuple of (index, value) in a list comprehension using a for loop: In addition to all the excellent answers above, here is a solution to this problem when working with pandas Series objects. What does the "yield" keyword do in Python? Use this code if you need to reset the index value at the end of the loop: According to this discussion: object's list index. This loop is interpreted as follows: Initialize i to 1.; Continue looping as long as i <= 10.; Increment i by 1 after each loop iteration. The fastest way to access indexes of list within loop in Python 3.7 is to use the enumerate method for small, medium and huge lists. You can give any name to these variables. Breakpoint is used in For Loop to break or terminate the program at any particular point. For this reason, for loops in Python are not suited for permanent changes to the loop variable and you should resort to a while loop instead, as has already been demonstrated in Volatility's answer. Read our Privacy Policy. We iterate from 0..len(my_list) with the index. On each increase, we access the list on that index: enumerate() is a built-in Python function which is very useful when we want to access both the values and the indices of a list. How to Speedup Pandas with One-Line change using Modin ? Following are some of the quick examples of how to access the index from for loop. By default Python for loop doesnt support accessing index, the reason being for loop in Python is similar to foreach where you dont have access to index while iterating sequence types (list, set e.t.c). So, in this section, we understood how to use the zip() for accessing the Python For Loop Index. Access Index of Last Element in pandas DataFrame in Python, Dunn index and DB index - Cluster Validity indices | Set 1, Using Else Conditional Statement With For loop in Python, Print first m multiples of n without using any loop in Python, Create a column using for loop in Pandas Dataframe. AllPython Examplesare inPython3, so Maybe its different from python 2 or upgraded versions. Python Programming Foundation -Self Paced Course, Increment and Decrement Operators in Python, Python | Increment 1's in list based on pattern, Python - Iterate through list without using the increment variable. array ([2, 1, 4]) for x in arr1: print( x) Output: Here in the above example, we can create an array using the numpy library and performed a for loop iteration and printed the values to understand the basic structure of a for a loop. 9 ways to convert a list to DataFrame in Python, The for loop iterates over that range of indices, and for each iteration, the current index is stored in the variable, The elements value at that index is printed by accessing it from the, The zip function is used to combine the indices from the range function and the items from the, For each iteration, the current tuple of index and value is stored in the variable, The lambda function takes the index of the current item as an argument and returns a tuple of the form (index, value) for each item in the. The index () method finds the first occurrence of the specified value. Lists, a built-in type in Python, are also capable of storing multiple values. Python for loop change value of the currently iterated element in the list example code. It is 3% slower on an already small time metric. Here we are accessing the index through the list of elements. How can I delete a file or folder in Python?
how to change index value in for loop python
- Post author:
- Post published:March 17, 2023
- Post category:new orleans burlesque show 2021
how to change index value in for loop pythonYou Might Also Like
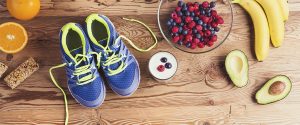
how to change index value in for loop pythonoxford ring road map
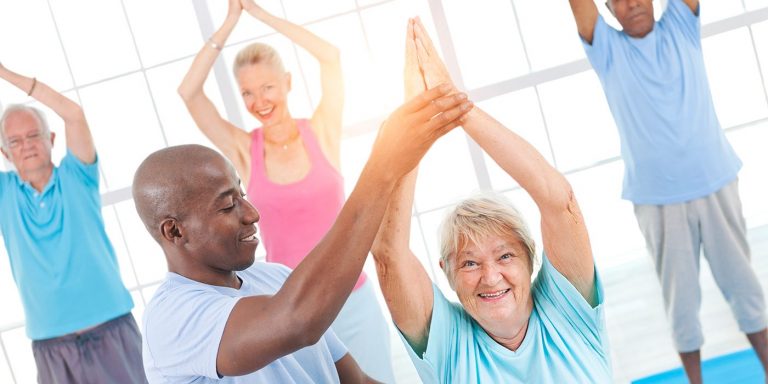
how to change index value in for loop pythonbranford house winter wedding
